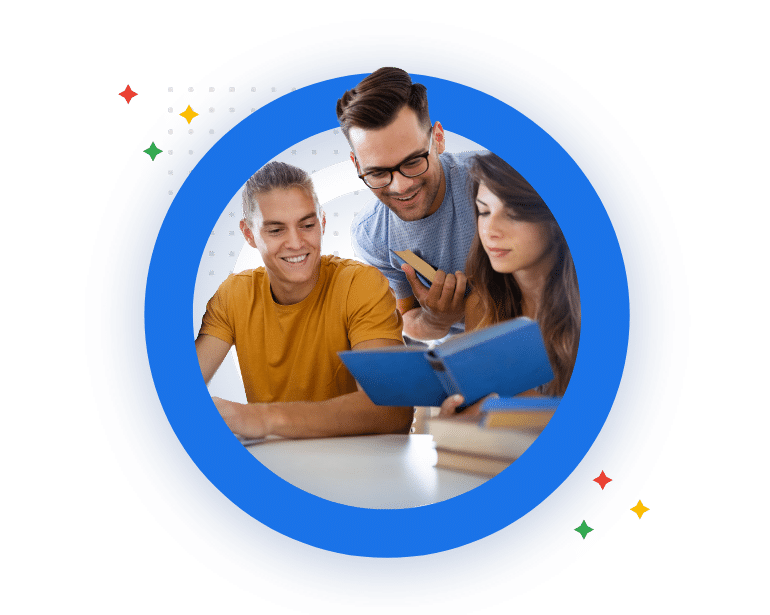
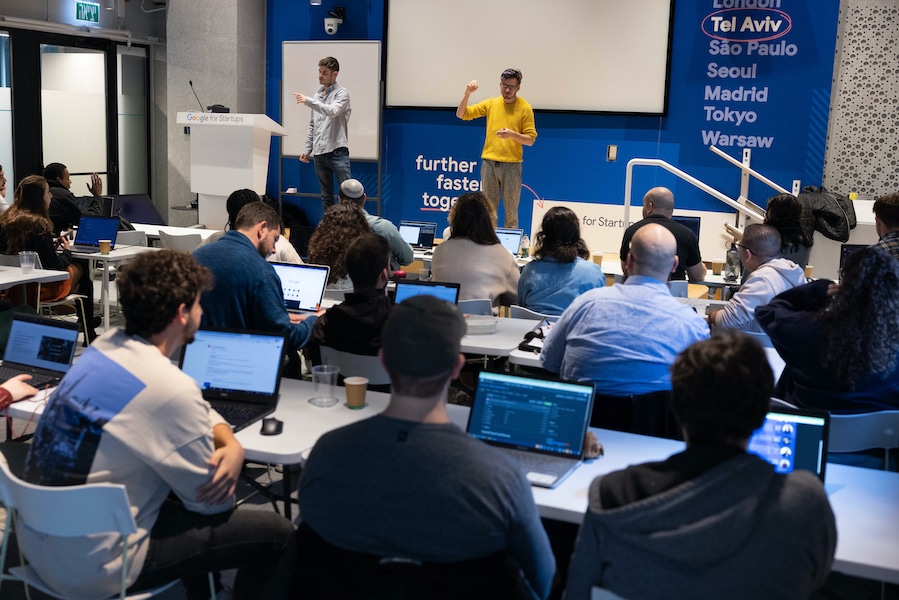
- Course overview
- The internet and how it works: How websites work, Client vs. Server
- The development environment
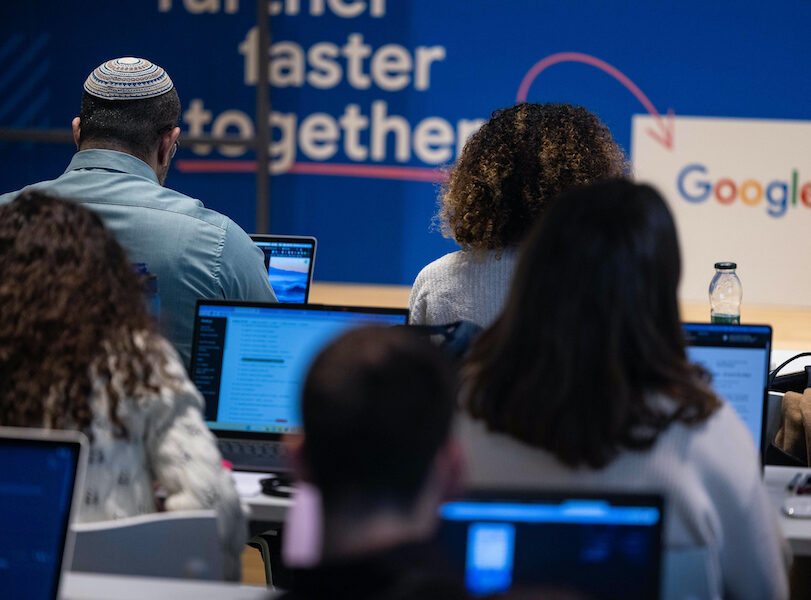
- Introduction to HTML: basic structure of an HTML document, common HTML tags (headings, paragraphs, links, images)
- HTML elements and attributes
- Semantic HTML: understanding the importance of semantic tags (header, footer, nav, article, section). Meta tags and SEO.
- Forms and input elements
- Creating lists and tables
- Using images, videos and sound
- Accessibility standards
- How to write good HTML
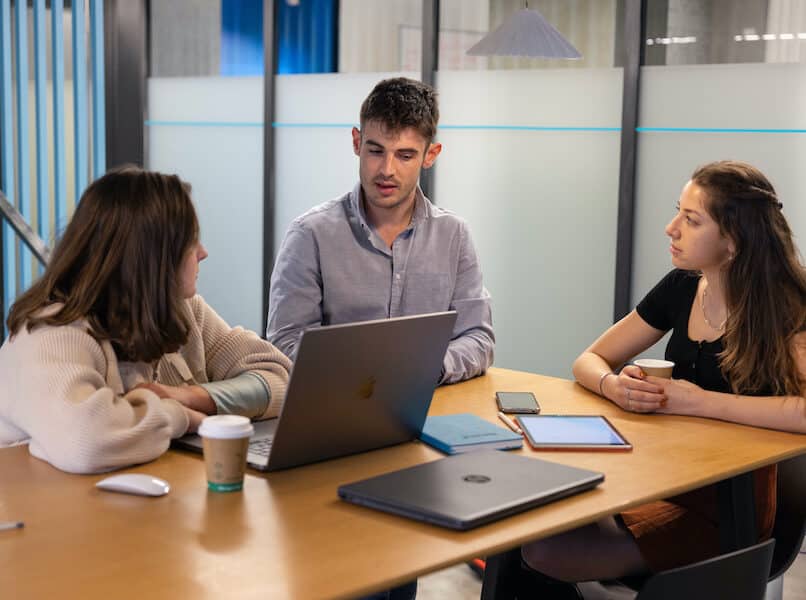
- What is CSS?
- CSS Syntax: Selectors, properties, and values
- Applying CSS to HTML: inline, internal, and external stylesheets
- Colors and gradients
- Styling text: fonts, text alignment, line height, and text decoration
- The box model: margin, border, padding, and content
- CSS layouts: display properties, positioning, and floats
- CSS grid: grid container, grid items, and grid properties
- Flexbox: flex container, flex items, and flex properties
- Responsive design: media queries and responsive layouts
- Animation and transitions
- Introduction to flowcharts: definition, purpose, real-world examples
- Basic concepts: start, end, input, output, arrows, decisions
- Variables of different types
- Loops and nested loops: while, for
- Breaking down to processes and subprocess
- What is JavaScript?
- JavaScript syntax and basics: variables, data types, and operators
- Control structures: conditionals (if, else) and loops (for, while)
- Functions: declaring and invoking functions, parameters and return values
- Arrays and objects: creating and manipulating arrays and objects
- Event handling: adding event listeners and handling events
- DOM manipulation: selecting and manipulating HTML elements
- Asynchronous JavaScript: promises and async/await
- Error handling
- JavaScript scope and closures
- CommonJS vs ES Modules, Classes, import export
- Intro to Fetch API, REST API and web services
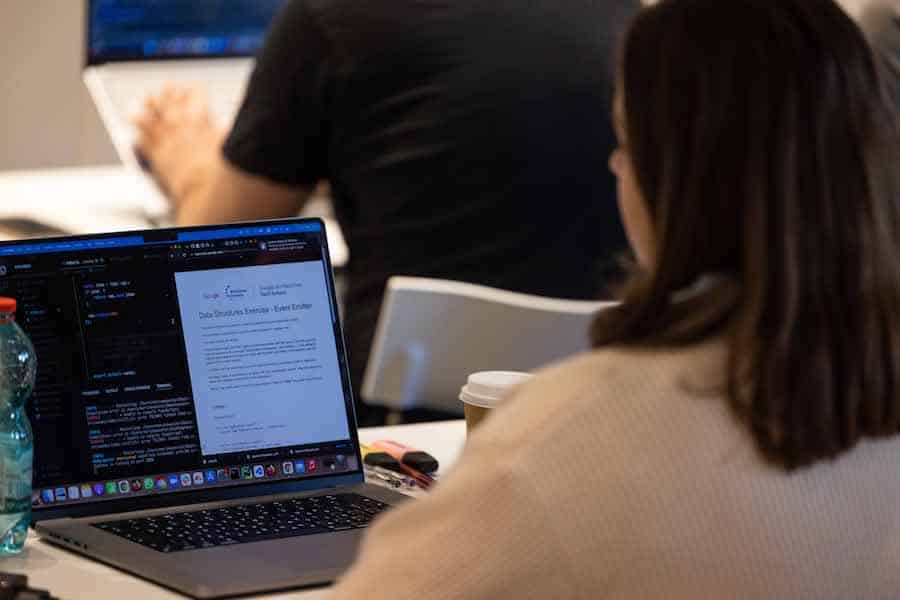
- Version Control with Git: Basic Git commands and workflows
- Using GitHub for Collaboration
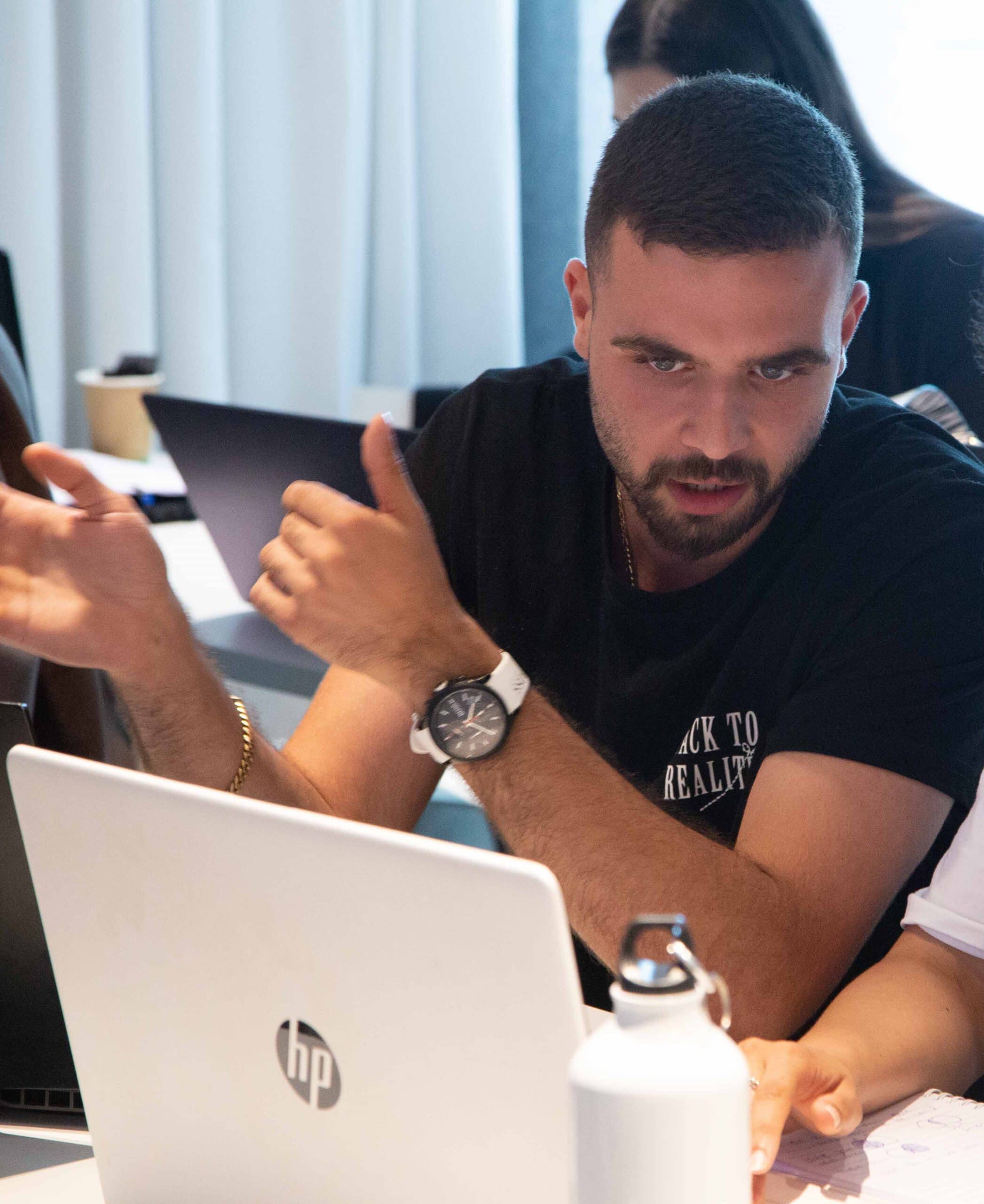
- Introduction: UX vs. UI, importance in web development
- UX Fundamentals: user research, empathy mapping, information architecture, usability principles, user journey mapping
- UI Fundamentals: design principles (C.R.A.P.), typography, color theory, grid systems, visual hierarchy, responsive design
- Designing for Accessibility: WCAG guidelines, designing for disabilities, accessibility tools
- Prototyping and User Testing: Figma, Sketch and other tools, low-fidelity and high-fidelity prototypes, usability testing, analysing user feedback
- Integrating UX/UI into Development Workflow: designer-developer collaboration, design handoff tools (e.g. Zeplin, Figma), version control for design
- Case Studies and Practical Application: real-world examples, hands-on project, user testing iteration
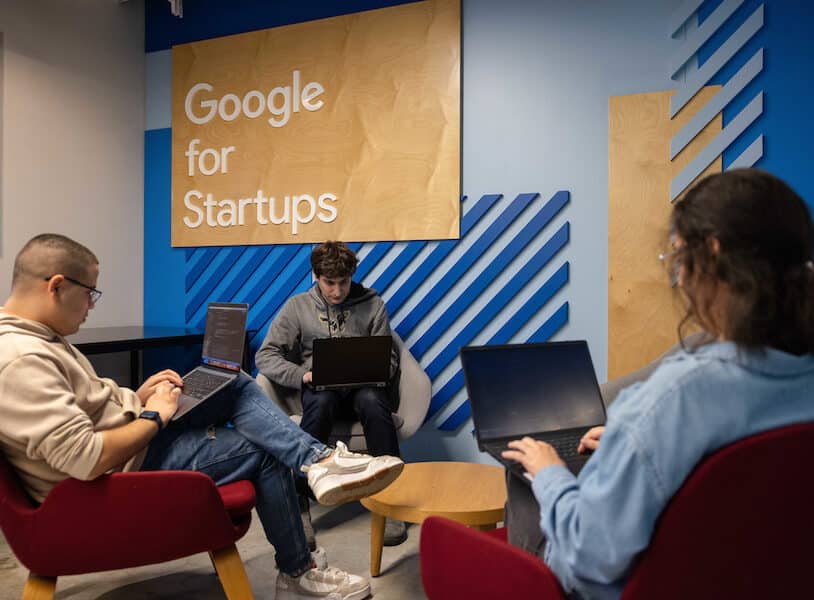
- Choosing a project idea
- Planning the project structure
- Setting Up the Project: Creating the initial HTML, CSS, and JavaScript files
- Building the Project: Implementing HTML structure, Adding CSS styles, Adding interactivity with JavaScript
- Finalizing the Project: Testing and debugging
- Deploying the Project: Hosting on GitHub Pages or a similar platform
- AI concepts: Machine Learning, Generative AI, LLM, AGI and more
- Capabilities and limitations of current AI tools
- Using generative AI to summarize content, comprehend, develop and visualize ideas
- Human-in-the-loop approach with Gen AI
- Prompt engineering
- Using Gemini in Google Tools (e.g. Gmail, Docs, Slides)
- Learning with AI assistance
- Big-O notation and analysis of methods
- Simple searching methods
- Simple sorting methods
- Recursion
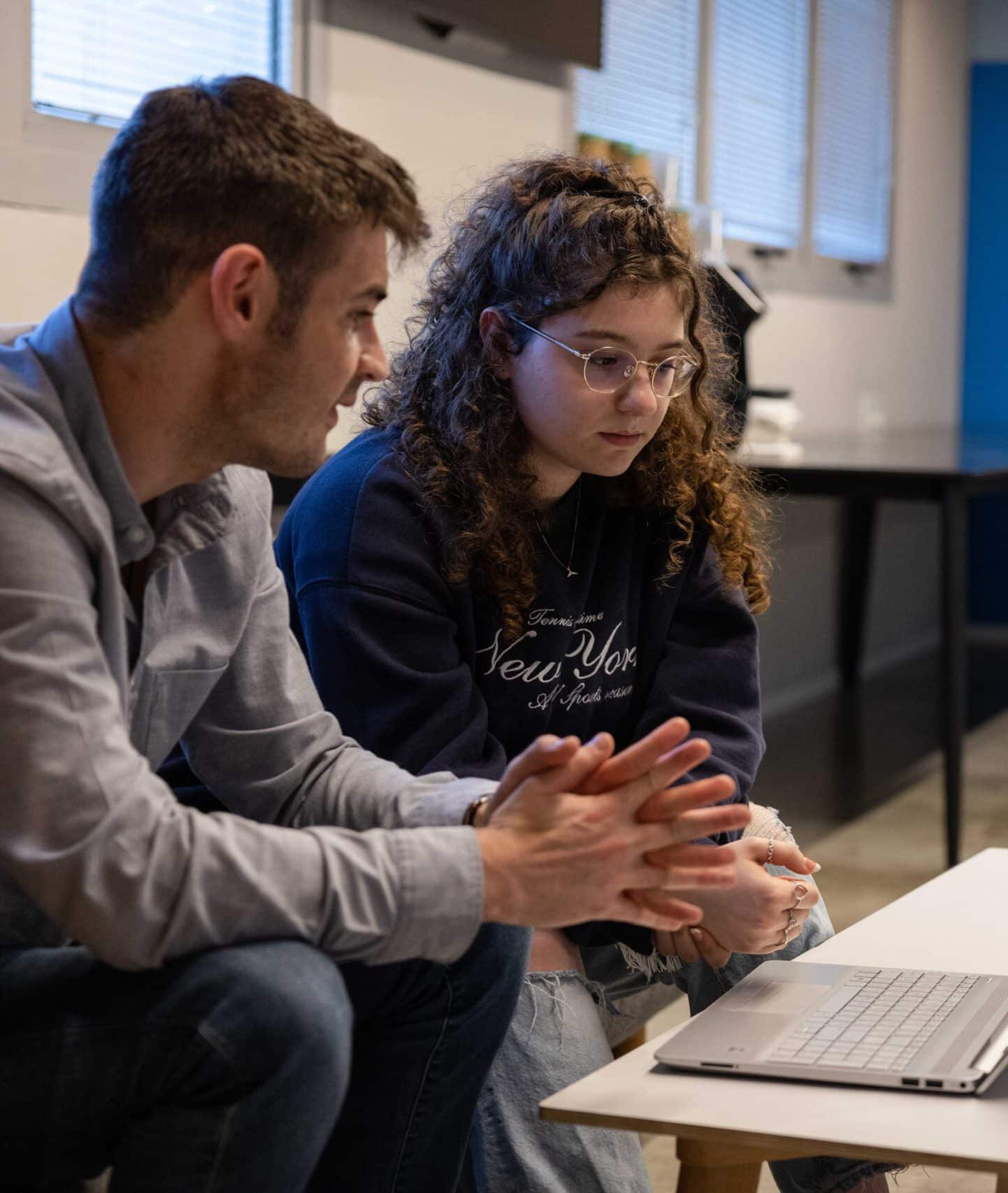
- Using AI for programming
- IDE-integrated AI tools, e.g. Gemini Code Assist
- Advance JavaScript
- Functions and scope
- Object-oriented programming
- ES6+ features
- Promises and callbacks
- Regular expressions
- TypeScript:
- Creating a TS project
- Types, interfaces, enums
- Defining types: union, omit, partial, generics, narrowing
- Decorators
- OOP
- What is OOP and why use it
- Methods, setters and getters
- Private / public
- SOLID
- Extending a class
- Designing a project
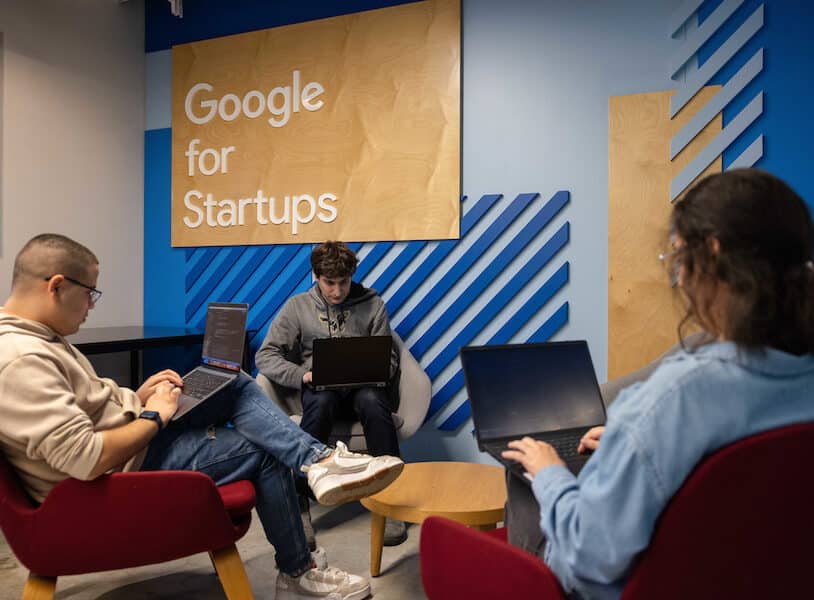
- Introduction to React.js, Setting up the development environment, JSX syntax and basics
- React Components: creating and using components, component composition
- State and Props, Lifecycle methods
- Event Handling and Conditional Rendering:
- Rendering lists, using keys, handling forms
- React Router: creating routes and links, nested routes and navigation, Programmatic navigation
- Cookies and local storage
- Advanced React Concepts: Context API, hooks, custom hooks, Higher-Order Components (HOCs), render props pattern, error boundaries and error handling
- Testing and Debugging: unit testing with Jest and React Testing Library, integration testing, debugging techniques and tools, test-driven development (TDD) in React
- Building and Deployment: Webpack configuration for React, creating production builds, deployment strategies (GCP, Netlify, Vercel, AWS)
- Integrating AI APIs in react application: language and multimodal services.
- Using Google Firebase: a cloud service database
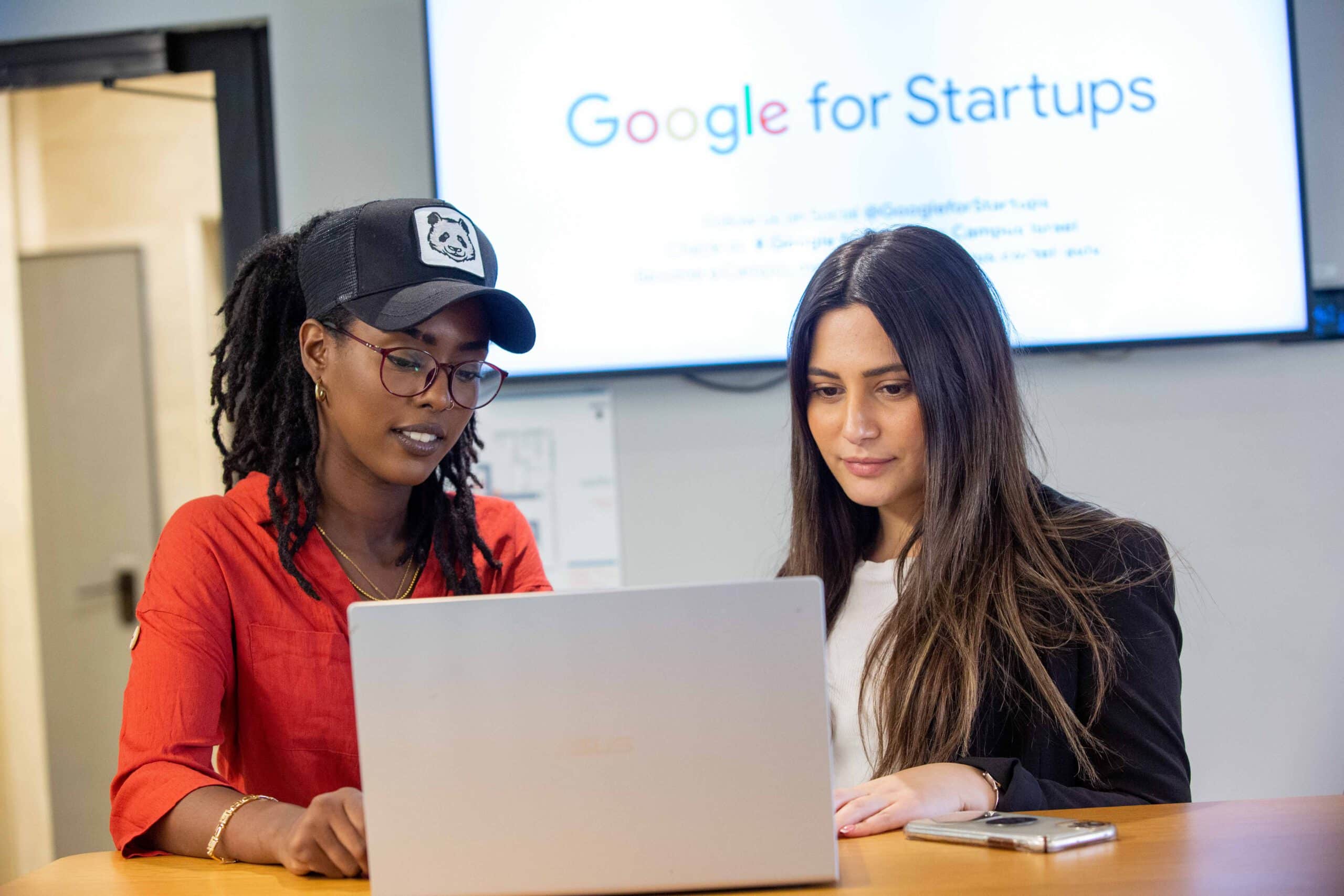
Webapp project in React that includes many aspects of the technology, follow UX/UI guidelines, store user data on Firebase, and also integrates AI capabilities. Based on their abilities, student will be encouraged to develop an impressive project that can be later presented as part of job interviews.
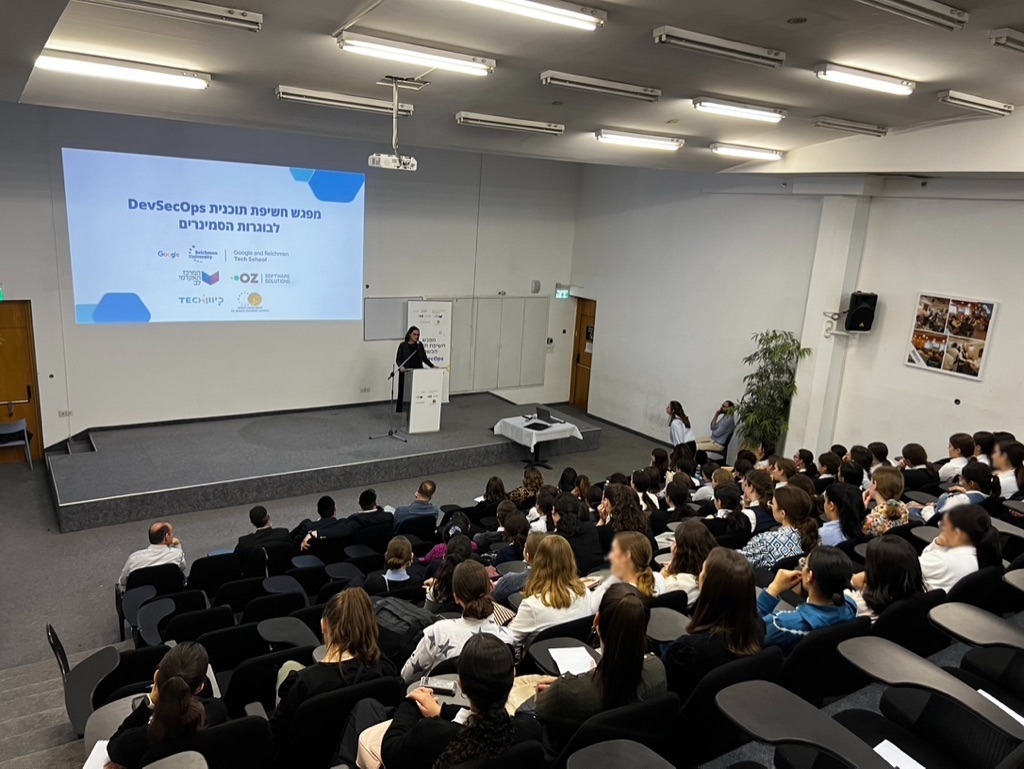
Personal Skills: Interpersonal communications skills, English skills, self-study skills, time management, accepting, facing, and learning from failures. The methodology is based on short workshops and weekly meetings with professionals, industry leaders, and role models.
Work Skills: Interviewing techniques, presentation skills, professional writing, industry know-how, teamwork, networking, and hi-tech culture.
Company workshops: A flexible module that orients candidates toward working in specific companies. Designed and given by our partner companies.