*changes may apply
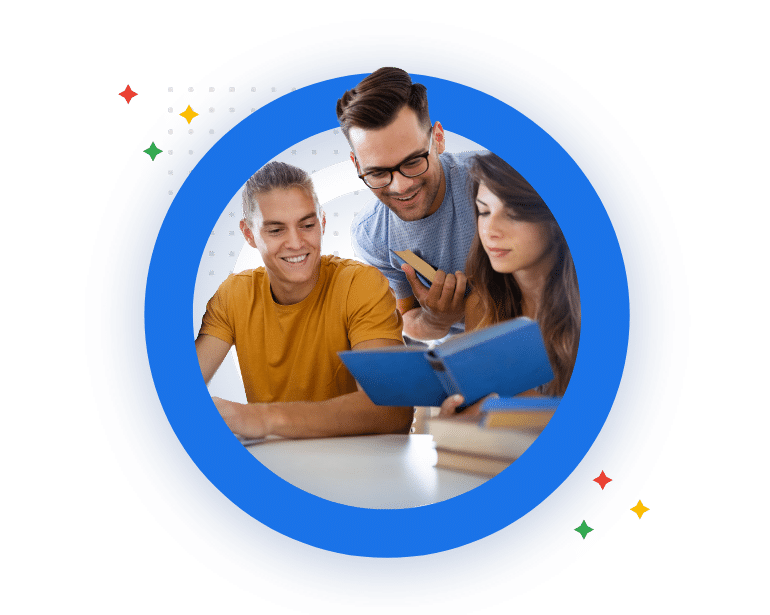
Detailed Syllabus
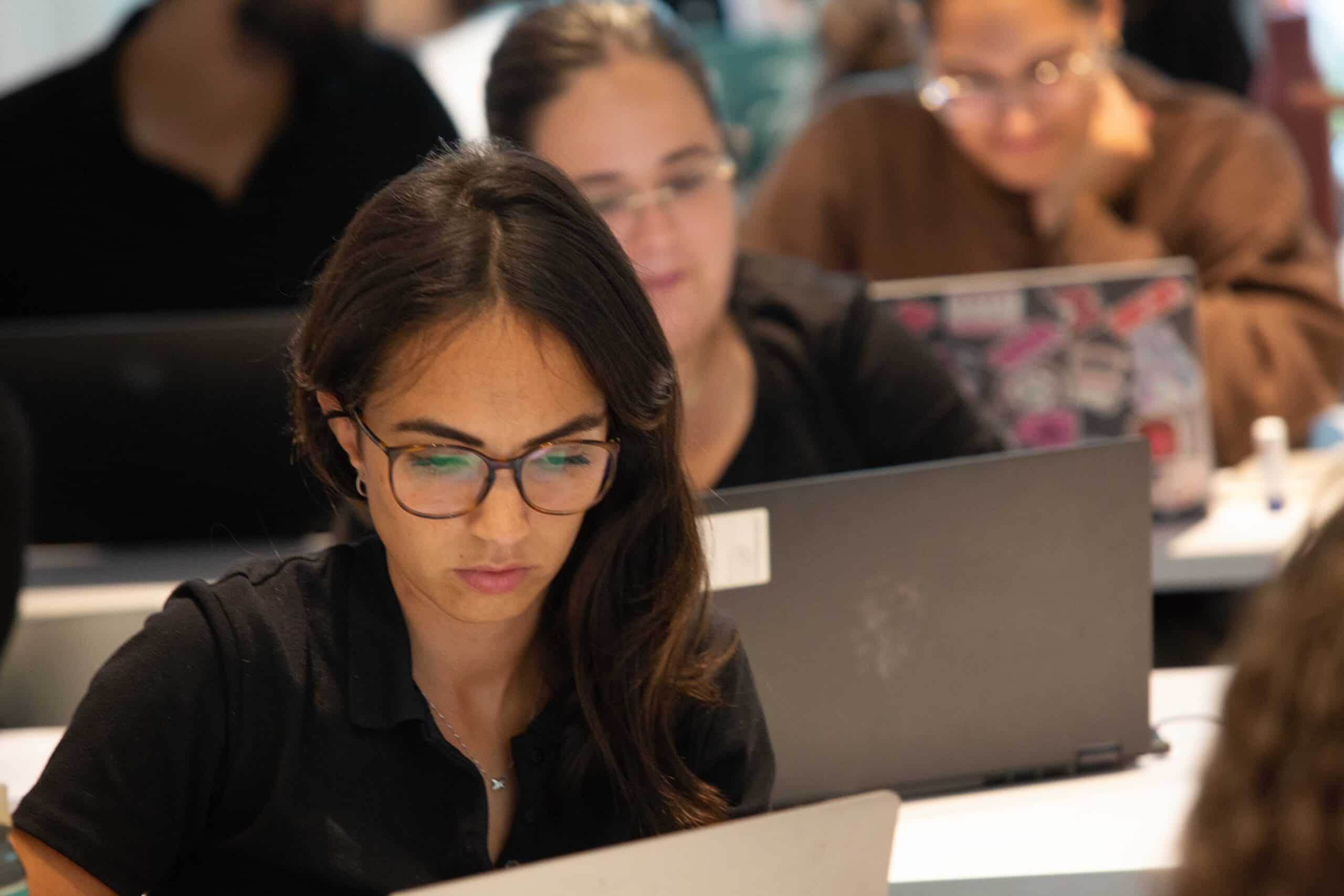
Android Jetpack Overview & Intro to Kotlin
- Introduction – Android Native Development
- Android OS Historical Overview
- AndroidX & Android Jetpack Components
- Android Platform Architecture
- JVM, DVM and ART
- Kotlin overview
- Kotlin’s Extensions
- Kotlin vals & vars
- Kotlin’s Nullability
- Kotlin Basics (if, when, loops, Arrays, Collections)
Kotlin – Functional Programming
- Functions & Lambdas
- Default Arguments
- High order Functions
- Collections high order functions (filter, map, flatMap…)
- Lazy Sequences
- Scope Functions
- infix functions
- extension functions
Object Oriented with Kotlin
- Classes – Constructors and Properties
- Inheritance
- Interfaces and Abstract classes
- Data classes
- Delegated properties & lazy initialization
- Object expressions and Object Declarations
- SAM interfaces
- Nested & inner classes
- Access modifiers and Generics
- Operator Overloading
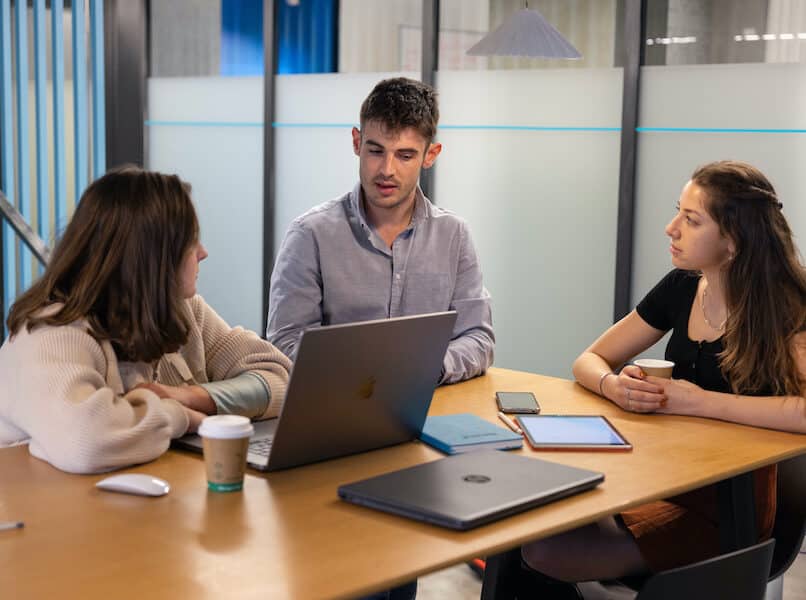
Android Studio with Gemini and Basic UI
- Intro to Android Studio and Gemini
- Creating projects and using AI Tools for code completion
- Using Emulators and physical Android OS devices
- App resource types and localization
- Basic Events handling and touch events
- Animations, Dialogs, and other basic UI components
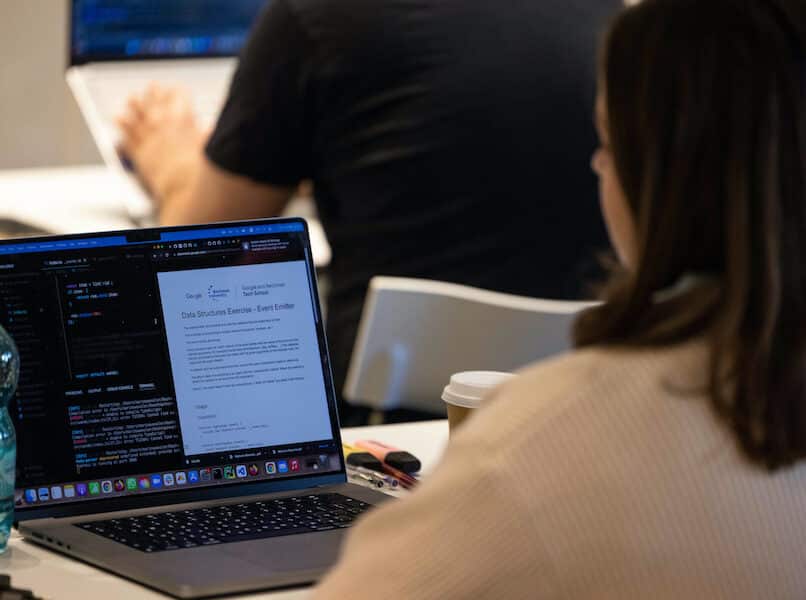
Android Building Blocks - Part 1
- Android App Components (Activity, Service, Receiver and Provider)
- Activities & Intents (Explicit and Implicit)
- Runtime Permissions using Launchers
- Activity Lifecycle and Shared preferences
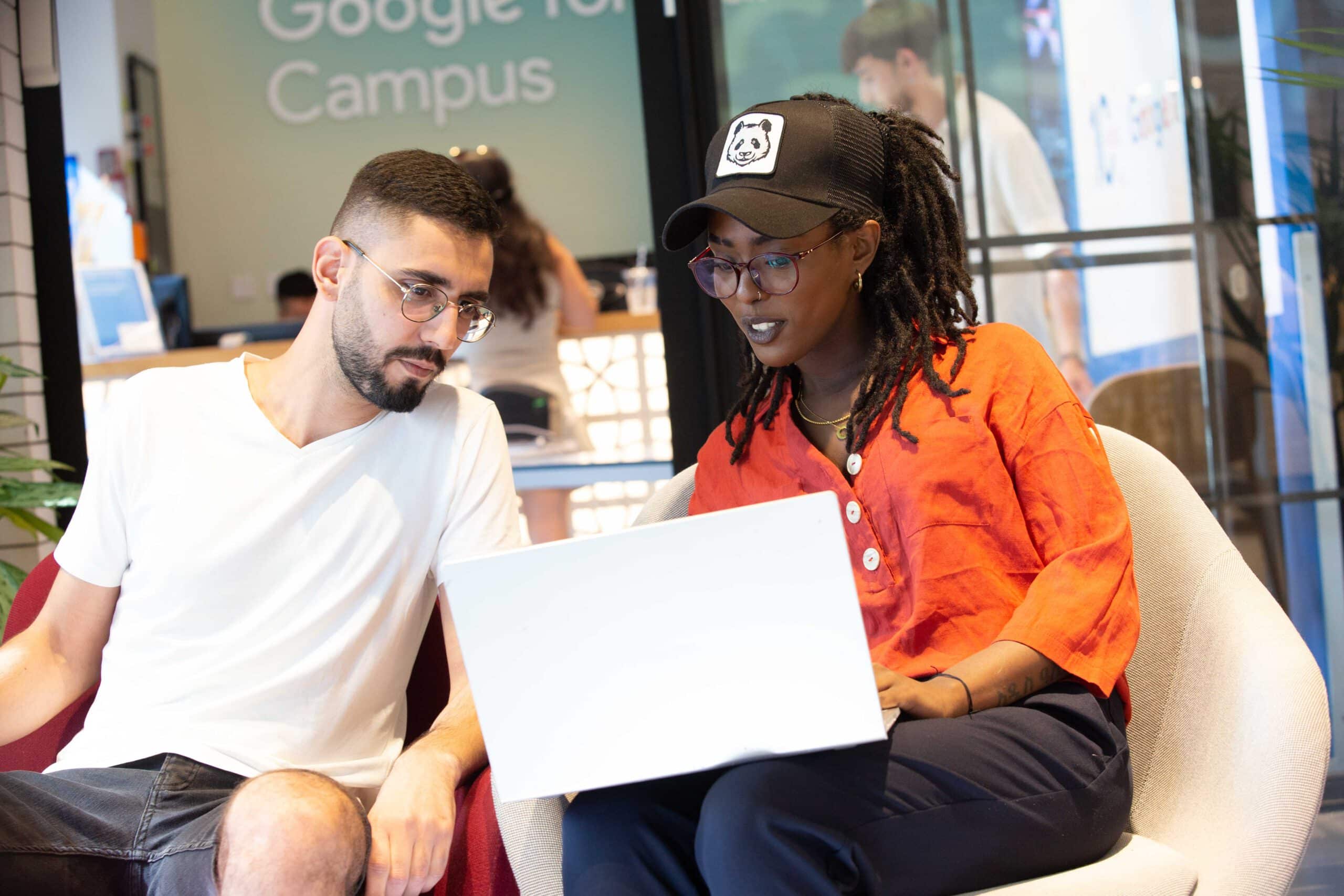
Android Building Blocks - Part 2
- Single Activity Architecture with Fragments
- Fragment Lifecycle
- Using Navigation for Fragments transactions
- Recycler View (Adapter, View Holder, Layout Manager)
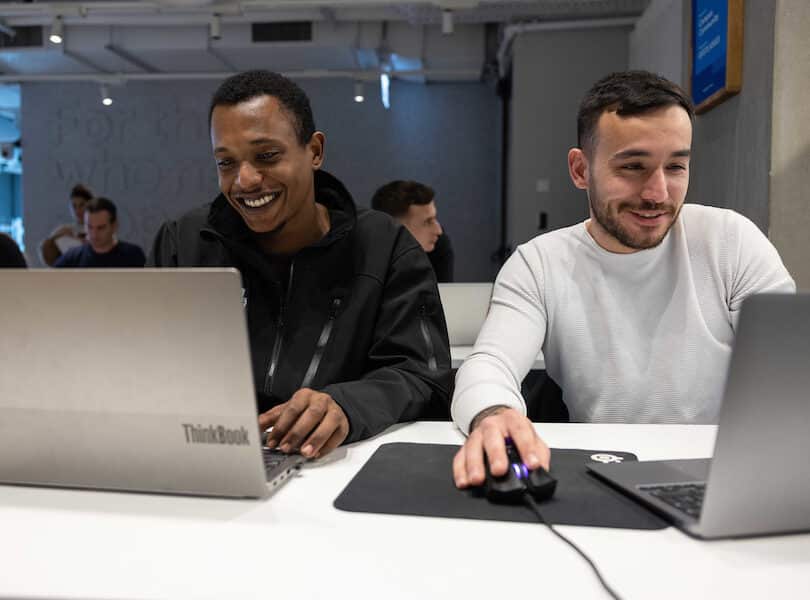
View Models & Live Data (MVVM)
- App Resources & Localization (language, screen, orientation…)
- Configuration changes, default UI behavior and legacy solutions
- MVVM Design pattern
- Jetpack Lifecycle object
- View Model Scope
- Live Data & Mutable Live Data
- Saved state module
- Transformations
- View Model provider Factory
MidProject using Room
- Internal Database with Room
- MVVM project with View Model, Live data, Navigation and Room.
Asynchronous programming with Coroutines
- Intro to Android Views and Bindings
- Making a Responsive UI
- Suspension over Blocking
- CoroutineScope & CoroutineContext
- Dispatchers
- Coroutines Builders (launch, async, withContext…)
- Combining Suspended Functions
- Jetpack Components Scopes
- Continuation Object
Phone services
- Launchers API for communication with system apps
- Working with the phone’s Camera
- Implementing speech recognition
- Android External Storage
- File Provider
- Google play services
- Fused location combined with Live Data
- Retrieving the user contacts
- Working with local phone databases
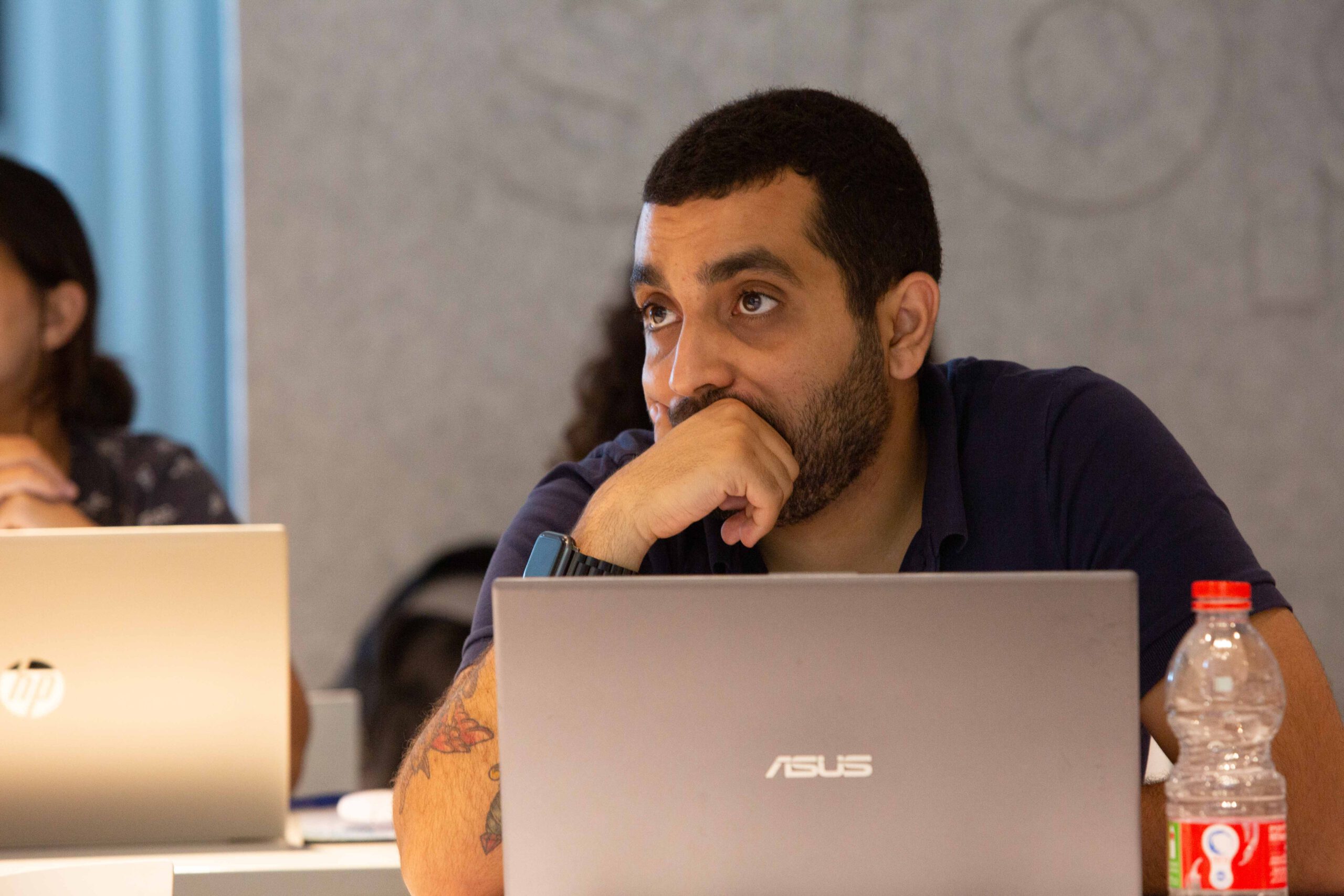
Firebase
- Working with Firebase Console
- Implementing Users Authentication
- Using Firebase Firestore for saving data on the cloud database
- Using Firebase Storage
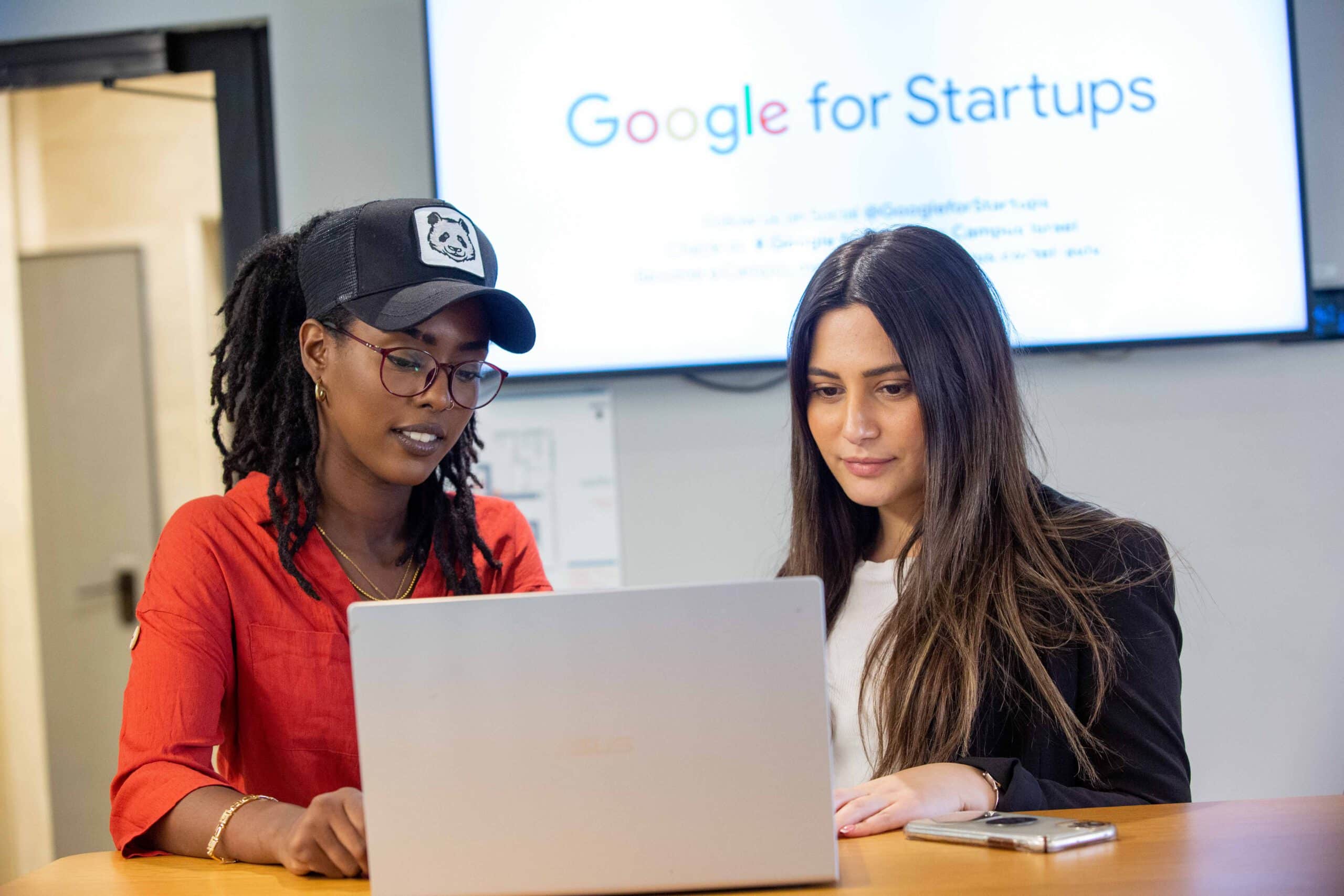
ML Kit
- Intro to Machine learning for mobile developers
- Choosing and integrating an API
- ML-Kit Android sample app
- Use-case 1: Text Recognition V2 API
- Use-case 2: Face Recognition API
- [Optional] OpenCV for computer vision
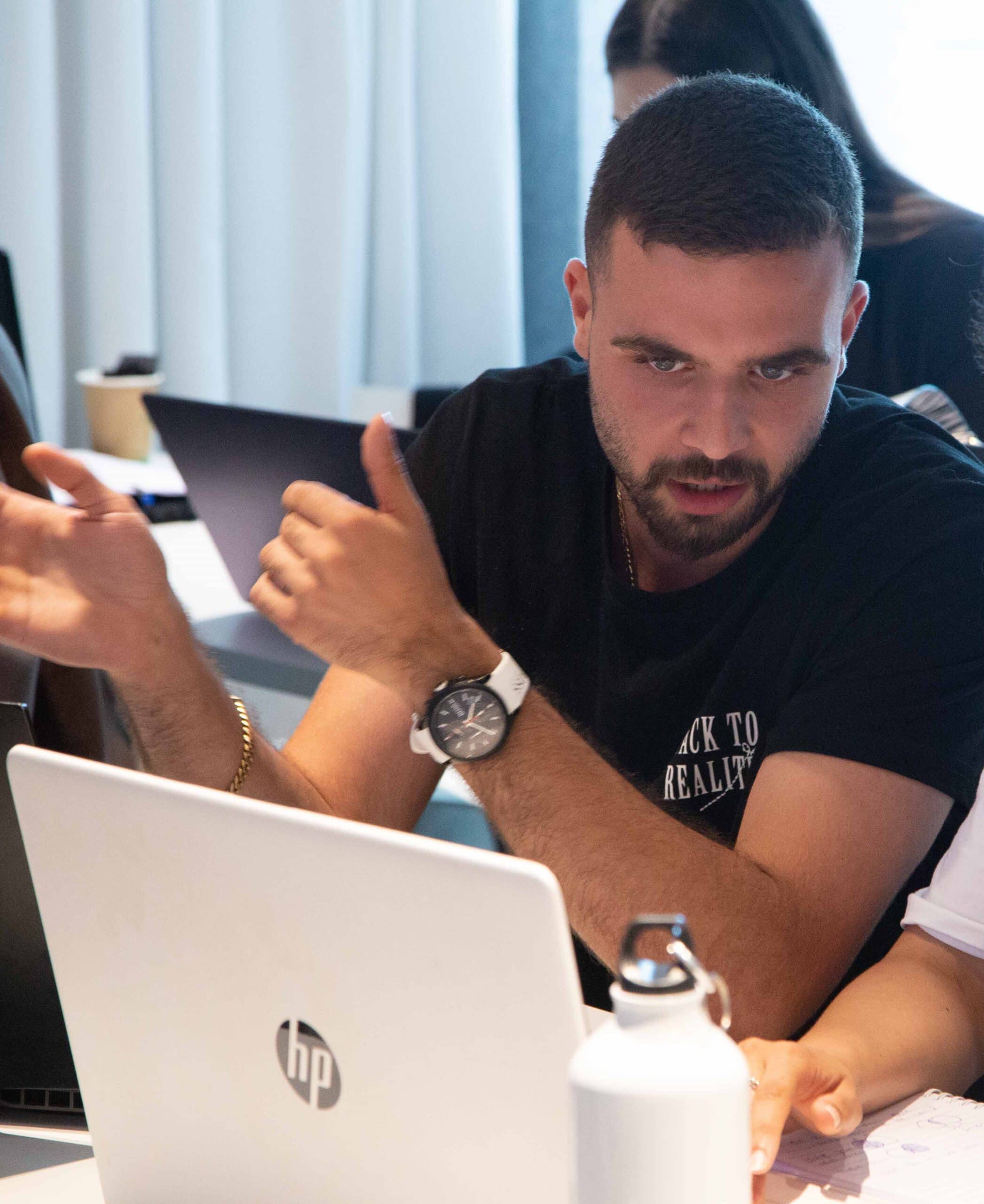
Components 1 – The Broadcasting system
- Notifications and PendingIntents
- Broadcast Receivers
- Static vs Dynamic Registration
- Local Broadcast Manager
- Alarm Manager
Components 2 – Non-UI Related tasks
- Service and Foreground service
- Job Schedulers
- Work Managers
- Background Execution limits
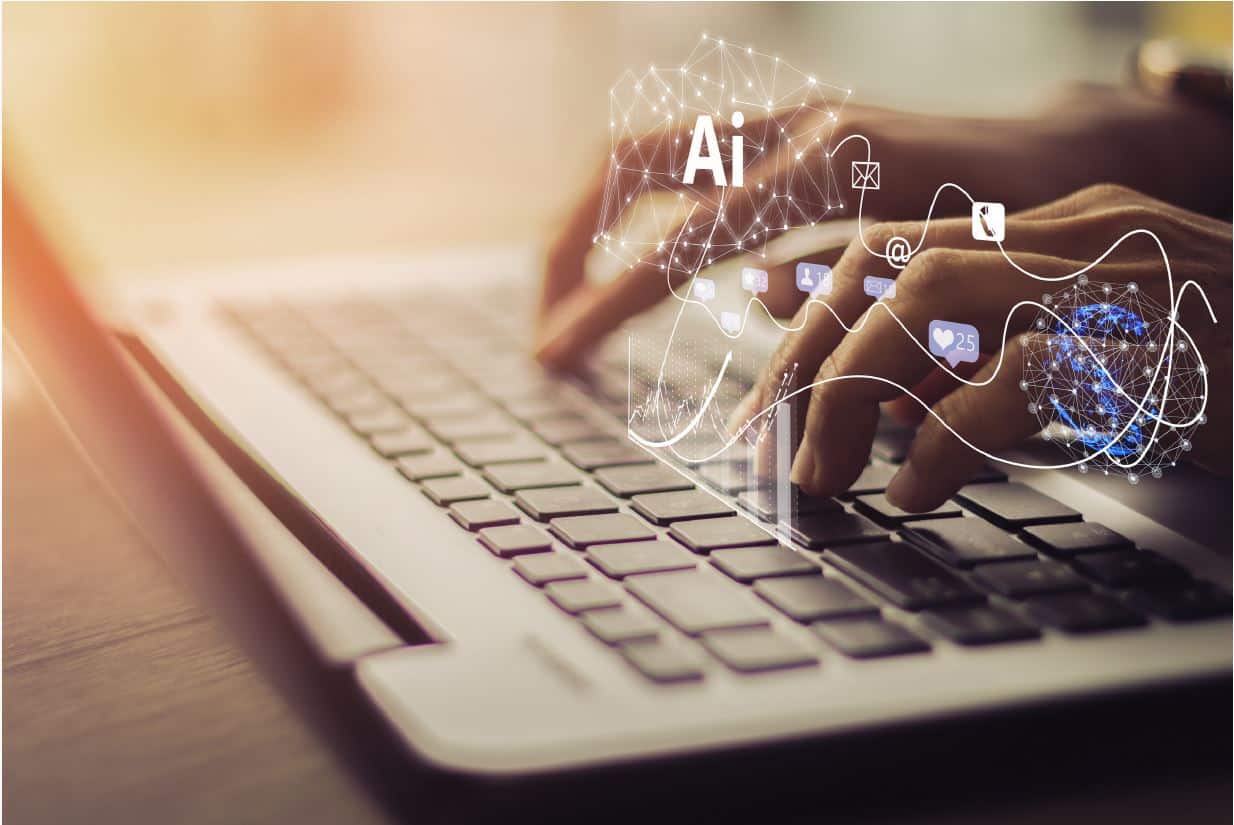
Jetpack Compise
- Introduction to Jetpack Compose: Overview of declarative UI & comparison with XML-based Views.
- Compose Basics: Understanding Composables, @Composable annotation, and creating simple UI elements.
- State Management: Introduction to state in Compose, using remember, mutableStateOf, and more
- Layouts in Compose: Exploring layout containers like Column, Row, Box…
- Modifiers: Using Modifier for styling, positioning, and adding behavior to Composables.
- Navigation in Compose: implementing navigation between Composables.
- Interoperability with Views: Iintegrating traditional Android Views within Compose and vice versa.
- Working with Lists: Implementing LazyColumn, LazyRow, and efficient scrolling lists.
- Handling Events and Side Effects: Understanding event handling in Compose and managing side effects with LaunchedEffect and SideEffect.
- Animations: Creating simple animations using animate* functions and AnimatedVisibility.